Send Telemetry to Lightstep from WebAssembly in the Browser
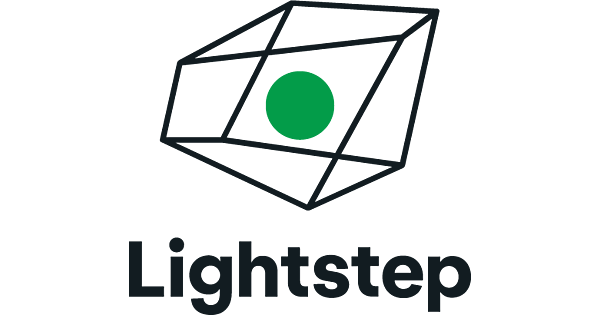
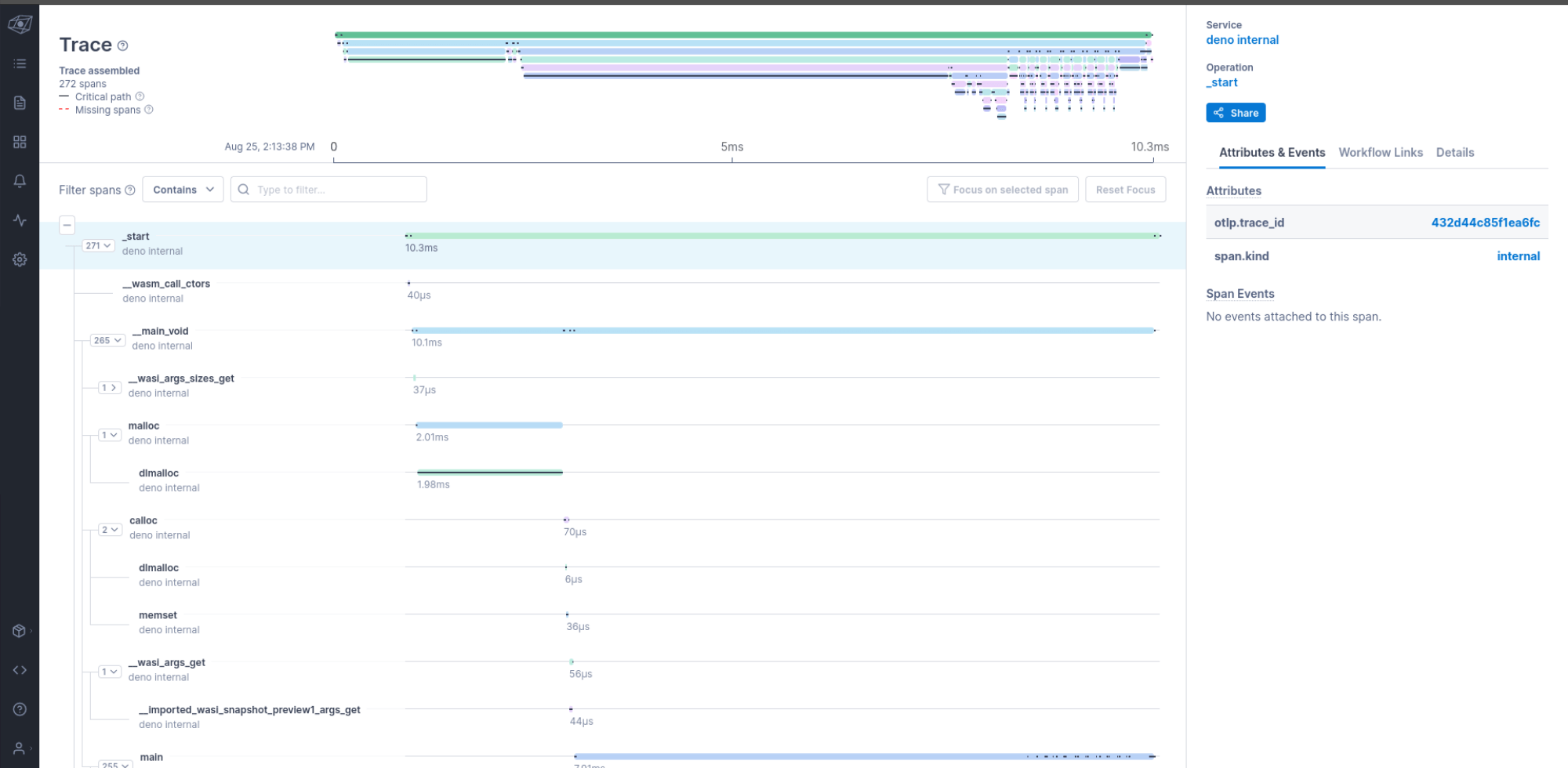
Overview
Enables the extraction of traces/spans from Wasm modules executing in a browser and emits them to Lightstep.
Installation
Install the Lightstep Adapter via npm
:
npm i @dylibso/observe-sdk-lightstep
Example
A basic web application that loads a Wasm module and executes it using the native WebAssembly runtime with a WASI browser shim. This assumes you are using a bundler such as esbuild or Webpack.
import { LightstepAdapter } from "@dylibso/observe-sdk-lightstep";
import { File, OpenFile, WASI } from "@bjorn3/browser_wasi_shim";
// setup some custom configuration for the adapter
const config = {
apiKey: "YOUR_API_KEY_HERE",
serviceName: "web",
emitTracesInterval: 1000,
traceBatchMax: 100,
host: "https://ingest.lightstep.com",
};
// create a new instance of the adapter with the config
const adapter = new LightstepAdapter(config);
// create an optional filter that instructs the adapter to throw away any
// spans below a configured threshold
const opts = {
spanFilter: {
minDurationMicroseconds: 100,
},
};
// fetch a wasm module and load up the bytes
const resp = await fetch("count_vowels.instr.wasm");
const bytes = await resp.arrayBuffer();
// start the adapter with the wasm module bytes and options
const traceContext = await adapter.start(bytes, opts);
// setup some files for stdin, stdout, and stderr
let fds = [
new OpenFile(
new File(
new TextEncoder("utf-8").encode(`count these vowels for me please`),
),
), // stdin
new OpenFile(new File([])), // stdout
new OpenFile(new File([])), // stderr
];
// instantiate the wasm module
let wasi = new WASI([], [], fds);
const instance = await WebAssembly.instantiate(bytes, {
"wasi_snapshot_preview1": wasi.wasiImport,
...traceContext.getImportObject(),
});
// execute the module
wasi.start(instance.instance);
let utf8decoder = new TextDecoder();
console.log(utf8decoder.decode(fds[1].file.data));
// instruct the adapter to stop the trace
traceContext.stop();
Adapter Configuration
You may modify the behavior of your adapter by passing in a configuration when initializing the adapter. A configuration has the following fields:
const config = {
// the URL of your OpenTelemetry collector
host: "https://ingest.lightstep.com",
// your Lightstep API key
apiKey: "YOUR_API_KEY_HERE",
// the dataset to group your observability data under
dataset: "web",
// how often to send new traces
emitTracesInterval: 1000,
// the maximum number of traces to send per request
traceBatchMax: 100,
};
const adapter = new LightstepAdapter(config);