Send Telemetry to Lightstep from WebAssembly in Deno
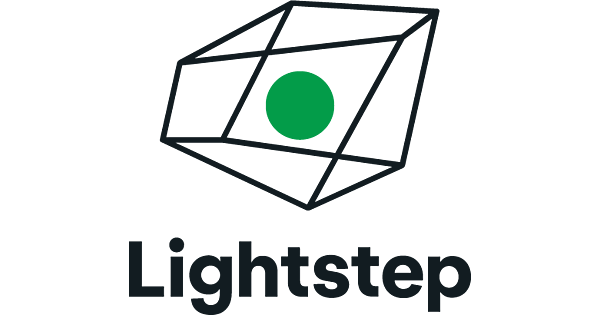
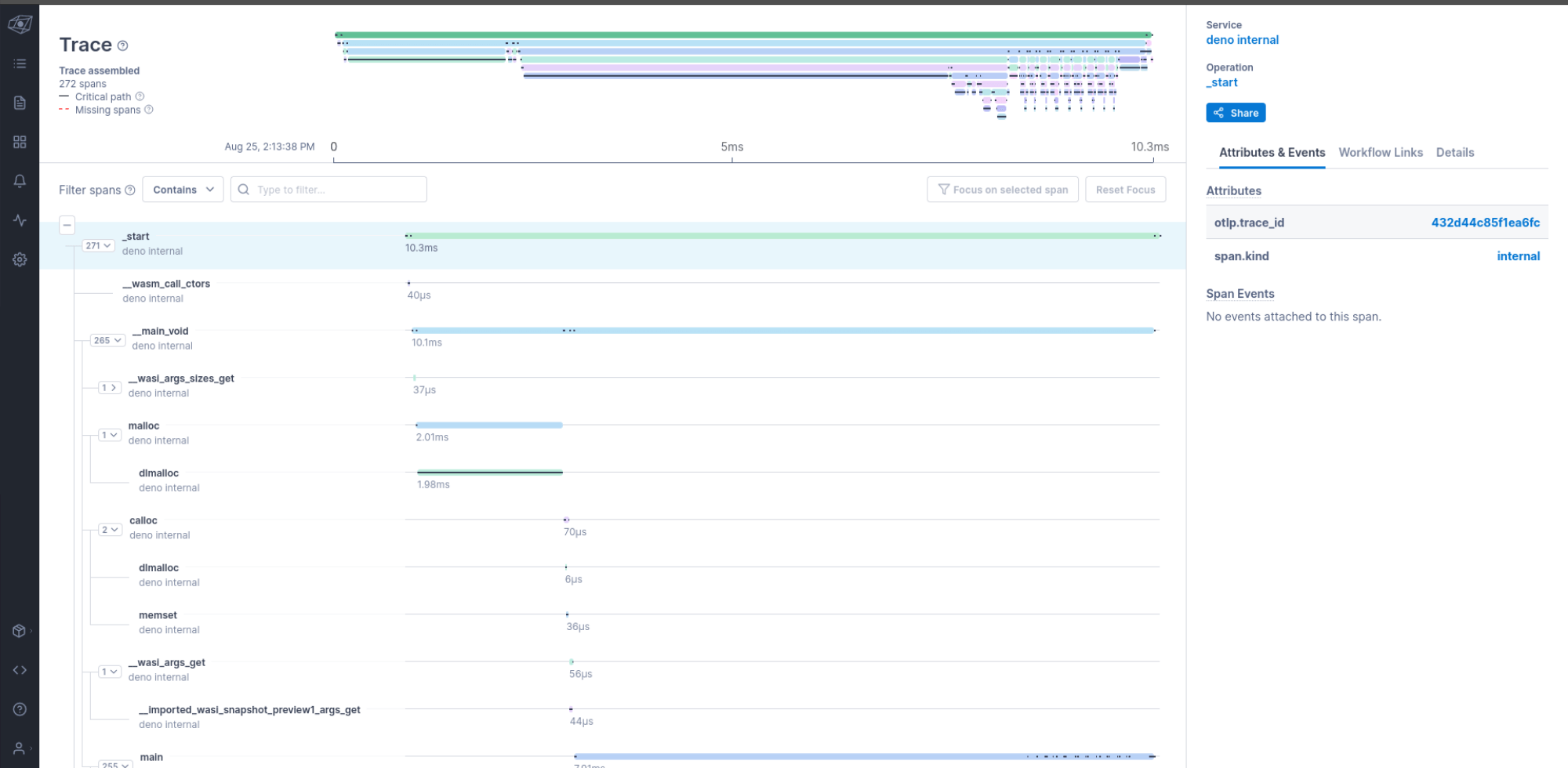
Overview
Enables the extraction of traces/spans from Wasm modules executing in a Deno environment and emits them to Lightstep.
Installation
Deno will install the package if it detects that you've imported it in your source code. See the example below.
Example
A basic Typescript program that loads a Wasm module and executes it using the native WebAssembly runtime.
import lightstep from "npm:@dylibso/observe-sdk-lightstep";
const {
LightstepAdapter,
LightstepConfig,
} = lightstep;
import Context from "https://deno.land/std@0.192.0/wasi/snapshot_preview1.ts";
import { load } from "https://deno.land/std/dotenv/mod.ts";
// setup some confgiruation and create a new instance of the Lightstep adapter
const env = await load();
const apiKey = env["LIGHTSTEP_API_KEY"];
const config: LightstepConfig = {
apiKey: apiKey,
serviceName: "deno",
emitTracesInterval: 1000,
traceBatchMax: 100,
host: "https://ingest.lightstep.com",
};
const adapter = new LightstepAdapter(config);
// load up a Wasm module
const bytes = await Deno.readFile("your_module.wasm");
// create an optional filter that instructs the adapter to throw away any
// spans below a configured threshold
const opts = {
spanFilter: {
minDurationMicroseconds: 100,
},
};
// start the adapter with the Wasm module bytes and options
const traceContext = await adapter.start(bytes, opts);
// load, instantiate and run the Wasm module
const module = new WebAssembly.Module(bytes);
const runtime = new Context({
stdin: Deno.stdin.rid,
stdout: Deno.stdout.rid,
});
const instance = new WebAssembly.Instance(
module,
{
"wasi_snapshot_preview1": runtime.exports,
...traceContext.getImportObject(),
},
);
runtime.start(instance);
// instruct the adapter to stop the trace
traceContext.stop();
Adapter Configuration
You may modify the behavior of your adapter by passing in a configuration when initializing the adapter. A configuration has the following fields:
const config = {
// the URL of your OpenTelemetry collector
host: "https://ingest.lightstep.com",
// your Lightstep API key
apiKey: "YOUR_API_KEY_HERE",
// the dataset to group your observability data under
dataset: "deno",
// how often to send new traces
emitTracesInterval: 1000,
// the maximum number of traces to send per request
traceBatchMax: 100,
};
const adapter = new LightstepAdapter(config);