Send Telemetry to Honeycomb from WebAssembly in Node.js
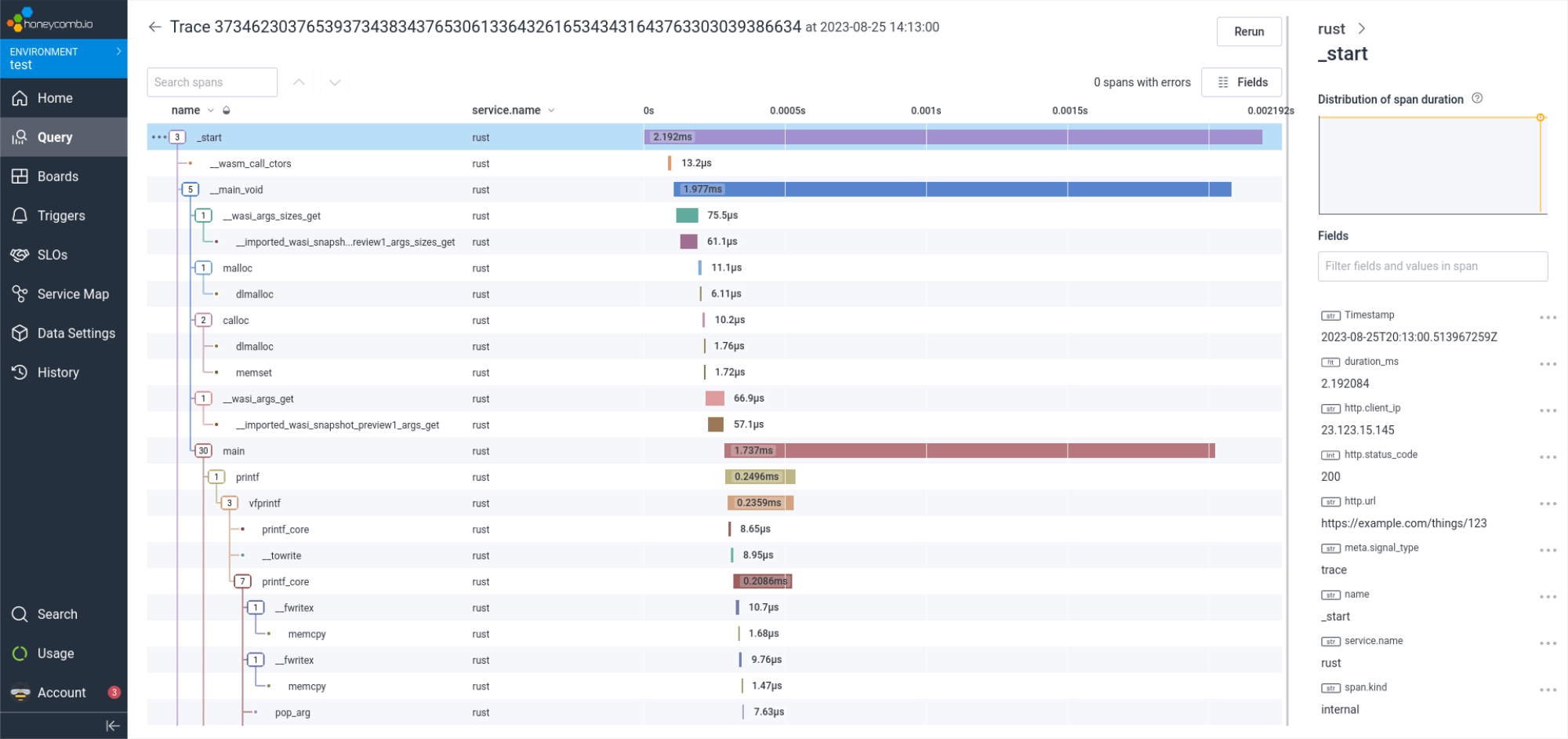
Overview
Enables the extraction of traces/spans from Wasm modules executing in a Node.js environment and emits them to Honeycomb.io.
Installation
Install the Honeycomb Adapter via npm
:
npm i @dylibso/observe-sdk-honeycomb
Example
A basic JavaScript program that loads a Wasm module and executes it using the native WebAssembly runtime.
const fs = require("fs");
const { WASI } = require("wasi");
const { env, argv } = require("node:process");
const { HoneycombAdapter } = require("@dylibso/observe-sdk-honeycomb");
require("dotenv").config();
const wasi = new WASI({
version: "preview1",
args: argv.slice(1),
env,
});
// setup some configuration and create a new instance of the Honeycomb adapter
const config = {
apiKey: process.env.HONEYCOMB_API_KEY,
dataset: "node",
emitTracesInterval: 1000,
traceBatchMax: 100,
host: "https://api.honeycomb.io",
};
const adapter = new HoneycombAdapter(config);
// create an optional filter that instructs the adapter to throw away any
// spans below a configured threshold
const opts = {
spanFilter: {
minDurationMicroseconds: 100,
},
};
// load up a Wasm module
const bytes = fs.readFileSync("your_module.wasm");
// instruct the adapter to start the trace
adapter.start(bytes, opts).then((traceContext) => {
const module = new WebAssembly.Module(bytes);
// instantiate and execute the module
WebAssembly.instantiate(module, {
...wasi.getImportObject(),
...traceContext.getImportObject(),
}).then((instance) => {
wasi.start(instance);
// associate some helpful metadata with the trace
traceContext.setMetadata({
http_status_code: 200,
http_url: "https://example.com",
});
// instruct the adapter to stop the trace
traceContext.stop();
});
});
Adapter Configuration
You may modify the behavior of your adapter by passing in a configuration when initializing the adapter. A configuration has the following fields:
const config = {
// the URL of your OpenTelemetry collector
host: "https://api.honeycomb.io",
// your Honeycomb API key
apiKey: "YOUR_API_KEY_HERE",
// the dataset to group your observability data under
dataset: "node",
// how often to send new traces
emitTracesInterval: 1000,
// the maximum number of traces to send per request
traceBatchMax: 100,
};
const adapter = new HoneycombAdapter(config);